Functions
A function is a script/piece of code to be executed. Functions exist to allow custom logic/behavior to be introduced to an AI character. With functions, you can get an AI character to perform actions that don't come with the AI character out of the box. These actions can be almost anything, from sending messages to users to calling external APIs. Currently, only JavaScript (opens in a new tab) functions are supported. Functions can be executed during the lifecycle of a conversation, or called automatically by an AI character.
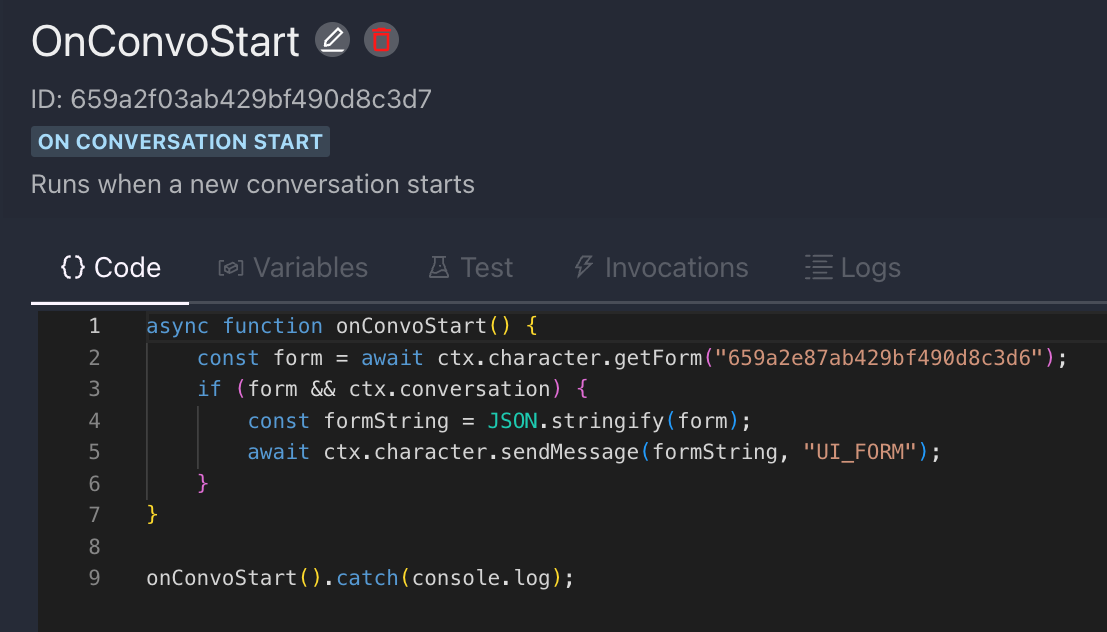
Sandbox
Functions run in safe sandboxes that have access to a few variables, some of which are:
ai
: An AI object that is used to send prompts/requests to various AI models.axios
: An instance ofAxiosStatic
from the popular axios (opens in a new tab) HTTP client.ctx
: An object that represents the current context in which the function is being executed.email
: An email client that is used to send emails.console
: This object provides access to the debugging console. Data written to the console can be found in the Logs tab.fetch
: A method that provides an easy, logical way to fetch resources asynchronously across the network. The fetch object is designed to match the Fetch API (opens in a new tab).fs
: A file system object that is used to create and access files.process
: This object provides information about the current Node.js process. The process object is a subset of the standard Process API (opens in a new tab), providing only theenv
property.- To see what environment your function is running in, read
process.env.NODE_ENV
. It's eitherdevelopment
orproduction
. - Your function's environment variables can also be accessed via
process.env
.
- To see what environment your function is running in, read
The code editor in the ProteusAI app comes with intellisense (opens in a new tab) which can help you figure out the members of variables, and the types of those members.
Triggers
A trigger is an event that causes a function to be executed. Supported triggers are:
ON_CONVERSATION_START
: The function gets executed when a new conversation starts.ON_MESSAGE_RECEIVED
: The function gets executed when an AI character receives a message.ON_MESSAGE_SENT
: The function gets executed when an AI character sends a message.ON_CHARACTER_CALL
: The function gets executed at the discretion of an AI character. This is a very powerful trigger as it gives an AI character the ability to perform actions based on its interaction with a user. For instance, if a user saysI'd like to send an email message saying "Good morning!"
, the AI character with which the user is chatting will search for functions with the trigger typeON_CHARACTER_CALL
whose names and parameters are seen to be appropriate for the action the user needs to be performed. The AI character would then execute this function which could either send such an email message immediately or present the user with an opportunity to confirm or cancel the request before proceeding.
Here's a function of type ON_CHARACTER_CALL
that can automatically create a product in an inventory app using an API service.
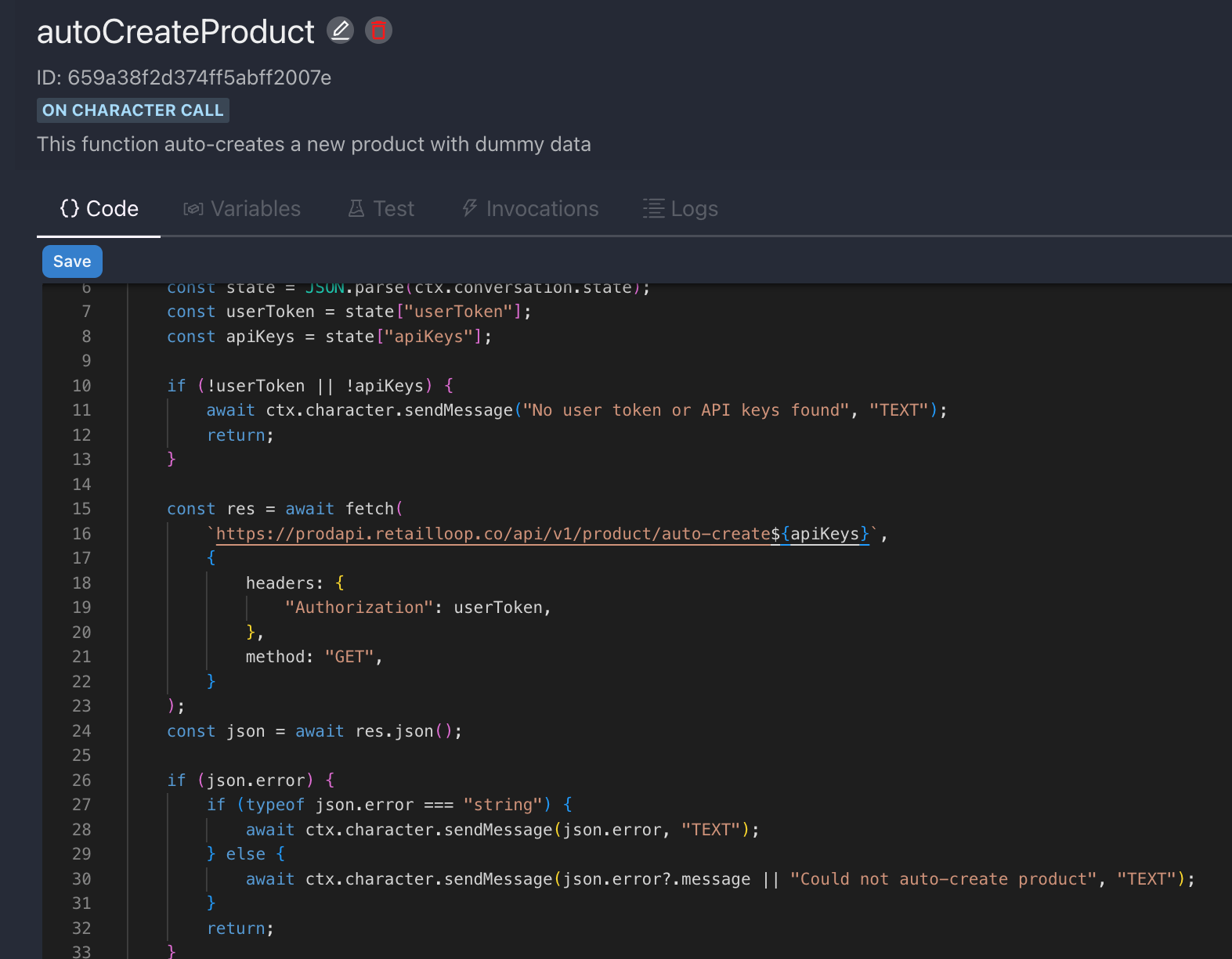
Now, having set up a connection to the API service, a user can simply ask the AI character to auto-create a product. The AI character can understand the user's request, recognize that there's a function that can be executed to serve that request, and then proceed to actually execute the function.
Parameters
Parameters define arguments that can be passed to a function when the function has a trigger type of ON_CHARACTER_CALL
. In ProteusAI, parameters are defined by adding entries
to the parameters object.
For instance, this is how you add a color
parameter to a function called changeBackgroundColor
.
When an AI character calls a function defined with parameters, it may put the args object into the ctx
object. The args
object will contain fields that match
the names of parameters, as well as values for those fields. This means that for the color
parameter, at runtime, you will be able to read the value of color
from ctx.args.color
. (See line 5.)
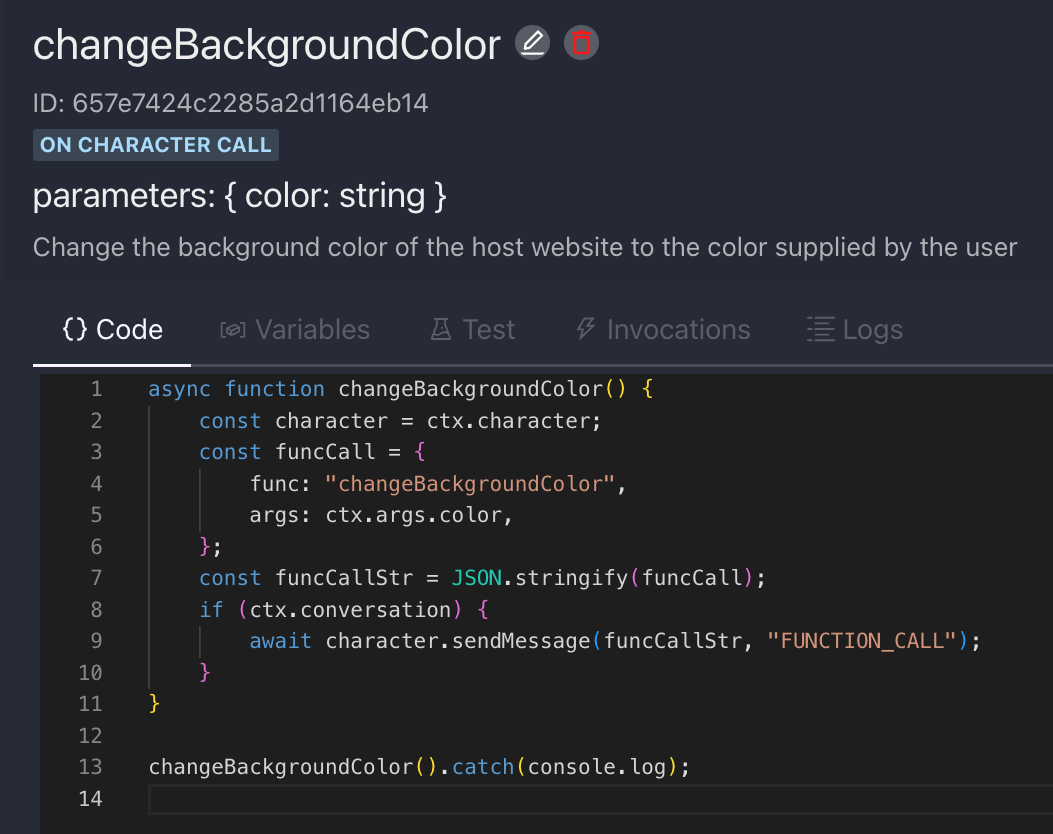
Here is the AI character calling the function at runtime, passing in the right color
argument.
If you expect a function to be invoked by a form submission, you can read the form data of the submitted form from ctx.formData.
For instance, you can get the value of the name
input field from ctx.formData.name
(or ctx.formData["name"]
).
Test tab
Coming soon!
This tab will allow you test your functions.
Variables tab
This tab allows you store a function's environment variables. Environment variables can be
accessed via process.env
during the function's runtime. For instance, the environment variable EMAIL_ADDRESS
can be read via process.env.EMAIL_ADDRESS
.
Environment variables that are marked as sensitive are not displayed on the user interface.
Environment variables are case-sensitive; URL
is different from url
.
Invocations tab
Whenever a function is run/executed, you get a function invocation. The Invocations tab lists the various invocations of a function.
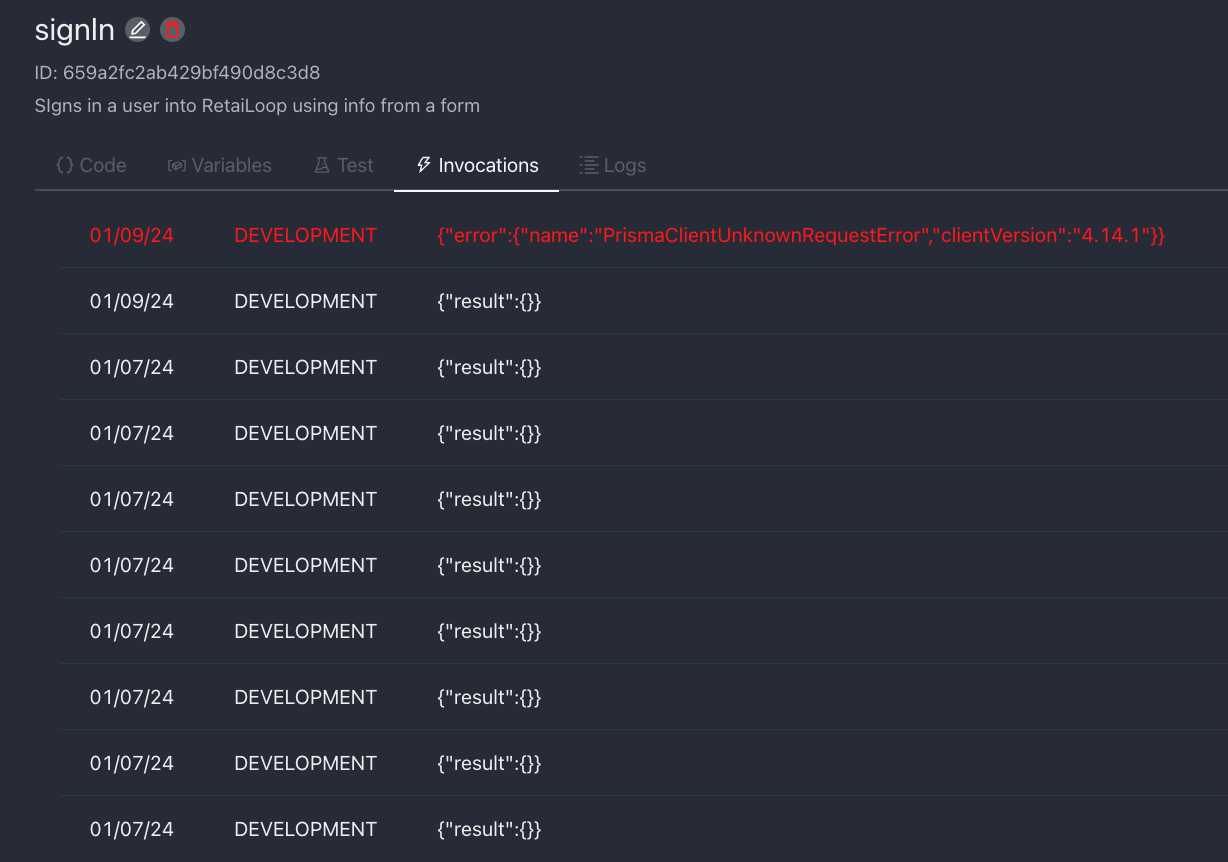
Logs tab
This tab shows logs produced by calling console.xxx
(e.g. console.log
, console.error
, etc.) during the execution of a function.
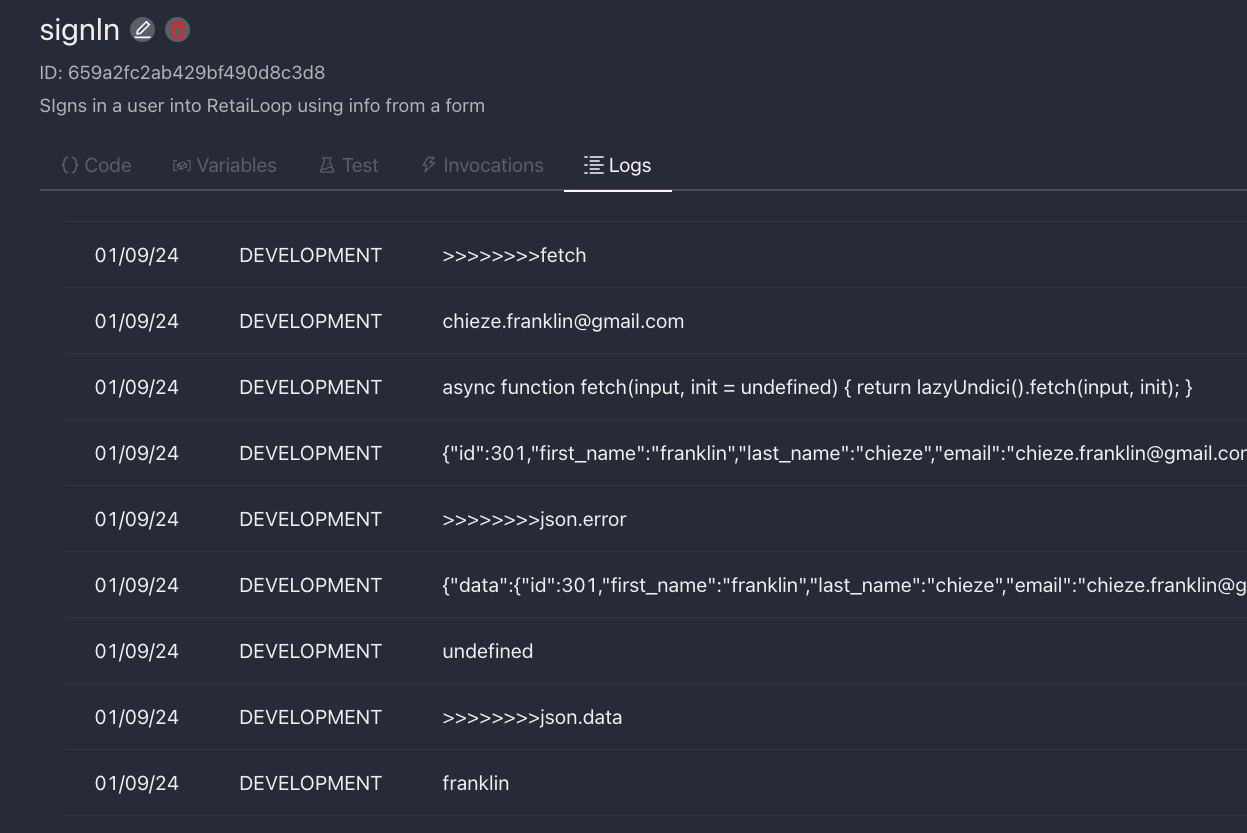